Add custom field to order admin woocommerce
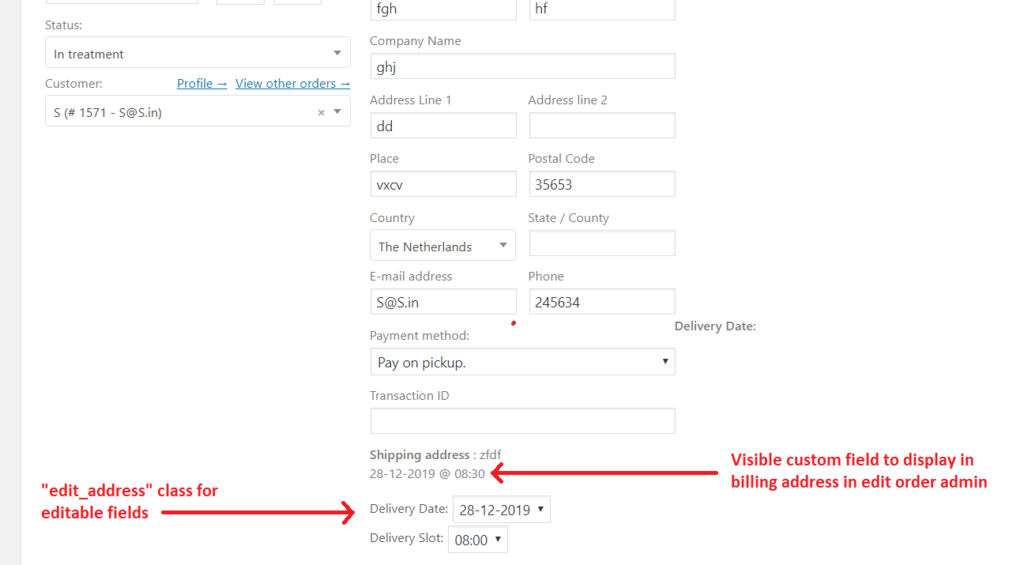
Above image shows where custom field will be added using code below.
1. In screenshot you will see editable fields that can be modified by admin. You will need to add a “edit_address” class as a wrapper for those fields to make them editable.
2. You can make a class “address” as a wrapper for displaying custom fields.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
/** | |
* Display field value on the order edit page | |
*/ | |
add_action( 'woocommerce_admin_order_data_after_billing_address', 'wdt_delivery_date_admin_order_meta', 10, 1 ); | |
function wdt_delivery_date_admin_order_meta($order){ | |
global $wpdb; | |
$strSelect = "SELECT meta_key FROM {$wpdb->prefix}postmeta WHERE post_id = '" . $order->get_id() . "' AND meta_key LIKE '_wdt_slot_%'"; | |
$arrSelect = $wpdb->get_results($strSelect); | |
list($date, $slot) = explode( '_', str_replace( '_wdt_slot_' . date('Y') . '_', '', $arrSelect[0]->meta_key)); | |
echo '<p class="address"><strong>'.__('Delivery Date').':</strong> <br/>' . $date . ' @ ' . $slot . '</p>'; | |
$arrData = get_option('_woo_delivery_slots'); | |
$arrOptions = $arrOptions2 = array(); | |
foreach ($arrData[date('Y')] as $key => $value) { | |
$flag_useful = false; | |
foreach ($value as $keyI => $valueI) { | |
if(isset($valueI['active']) && $valueI['active'] == 'true' && isset($valueI['value']) && intval($valueI['value']) > 0) { | |
$flag_useful = true; | |
} | |
} | |
if($flag_useful) { | |
$arrOptions[] = $key; | |
$arrOptions2[$key] = $key; | |
} | |
} | |
$arrOptions2 = array_reverse($arrOptions2); | |
$arrSlots = array(); | |
foreach ($arrData[date('Y')][$date] as $key => $value) { | |
$strCount = "SELECT count(*) used FROM {$wpdb->prefix}postmeta WHERE meta_key = '_wdt_slot_" . date('Y') . '_' . $date . "_" . $key . "' GROUP BY meta_key"; | |
$arrCount = $wpdb->get_results($strCount); | |
if(isset($value['active']) && $value['active'] == 'true' && ((!isset($arrCount[0]->used) || empty($arrCount[0]->used) || $arrCount[0]->used < $value['value']) || current_user_can( 'manage_options' ) ) && $value['value'] > 0) { | |
$arrSlots[$key] = $key; | |
} | |
} | |
?><div class="edit_address"><?php | |
echo 'Delivery Date : <select name="sel_myaccount_delivery_date" id="sel_myaccount_delivery_date">'; | |
foreach ($arrOptions2 as $key => $value) { | |
echo '<option value="' . $value . '" ' . ($value == $date?' selected="selected"':'') . '>' . $value . '</option>'; | |
} | |
echo '</select>'; | |
echo '<br/>Delivery Slot : <select name="sel_myaccount_delivery_slot" id="sel_myaccount_delivery_slot">'; | |
foreach ($arrSlots as $key => $value) { | |
echo '<option value="' . $value . '" ' . ($value == $slot?' selected="selected"':'') . '>' . $value . '</option>'; | |
} | |
echo '</select>'; | |
?></div><?php | |
} |